How do you find duplicates in a linked list?
Table of Contents
- How do you find duplicates in a linked list?
- Does linked list allow duplicates?
- How do I remove duplicates from a list?
- How do you remove alternate nodes from a linked list?
- How do you remove duplicates from an array?
- How HashSet remove duplicates from a list?
- Does linked list allow null values?
- How to remove duplicates from linked list in Java?
- How to remove duplicate elements from a list?
- How to create a list without duplicates in Java?
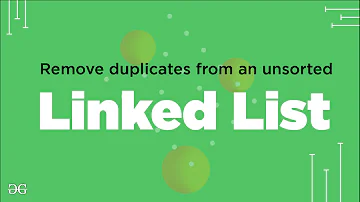
How do you find duplicates in a linked list?
Approach:
- Count the frequency of all the elements of the linked list using a map.
- Now, traverse the linked list again to find the first element from the left whose frequency is greater than 1.
- If no such element exists then print -1.
Does linked list allow duplicates?
A LinkedList can store the data by use of the doubly Linked list. ... The LinkedList can have duplicate elements because of each value store as a node.
How do I remove duplicates from a list?
Approach:
- Get the ArrayList with duplicate values.
- Create a new List from this ArrayList.
- Using Stream(). distinct() method which return distinct object stream.
- convert this object stream into List.
How do you remove alternate nodes from a linked list?
Given a Singly Linked List, starting from the second node delete all alternate nodes of it. For example, if the given linked list is 1->2->3->4->5 then your function should convert it to 1->3->5, and if the given linked list is 1->2->3->4 then convert it to 1->3.
How do you remove duplicates from an array?
Remove duplicates from sorted array
- Create an auxiliary array temp[] to store unique elements.
- Traverse input array and one by one copy unique elements of arr[] to temp[]. Also keep track of count of unique elements. Let this count be j.
- Copy j elements from temp[] to arr[] and return j.
How HashSet remove duplicates from a list?
The easiest way to remove repeated elements is to add the contents to a Set (which will not allow duplicates) and then add the Set back to the ArrayList : Set set = new HashSet(yourList); yourList. clear(); yourList.
Does linked list allow null values?
Null Elements: LinkedList allow any number of null values while LinkedHashSet also allows maximum one null element.
How to remove duplicates from linked list in Java?
There is another way to remove duplicates from linked list. Streams are introduced in java, so we can use the distinct () method of Stream. You can read the detailed working of the distinct () method. The distinct () method works based on Stream and returns a stream that contains only a unique element.
How to remove duplicate elements from a list?
Insert the elements of a list in the set one by one. Pick elements one by one. Compare the element with the rest of the elements. If you find a duplicate element then delete it. Else increment the pointer.
How to create a list without duplicates in Java?
Now we have a List without any duplicates, and it has the same order as the original List. If you like to have a function where you can send your lists, and get them back without duplicates, you can create a function and insert the code from the example above. Create a function that takes a List as an argument.